진행순서
1. djangorestframework 패키지 설치
2. user정보를 CRUD 할 수있는 API를 제공하는 api_user 앱 생성
3. REST API 설계
4. URLConf 설정을 통해 request 라우팅
5. view를 구현하여 request 처리
1. djangorestframework 패키지 설치
conda 명령어를 통해 djangorestframework를 설치하면 아래와 같이 패키지를 못찾는 경우가 있다.
$ conda install djangorestframework Collecting package metadata (current_repodata.json): done Solving environment: failed with initial frozen solve. Retrying with flexible solve. Collecting package metadata (repodata.json): done Solving environment: failed with initial frozen solve. Retrying with flexible solve. PackagesNotFoundError: The following packages are not available from current channels: - djangorestframework Current channels: - https://repo.anaconda.com/pkgs/main/osx-64 - https://repo.anaconda.com/pkgs/main/noarch - https://repo.anaconda.com/pkgs/r/osx-64 - https://repo.anaconda.com/pkgs/r/noarch To search for alternate channels that may provide the conda package you're looking for, navigate to https://anaconda.org and use the search bar at the top of the page. |
이런 경우 pip를 통해 설치해 준다.
$ pip install djangorestframework
Collecting djangorestframework
Downloading djangorestframework-3.11.0-py3-none-any.whl (911 kB)
2. user정보를 CRUD 할 수있는 API를 제공하는 api_user 앱 생성
아래의 명령어를 통해 api_user라는 이름을 같는 app을 생성한다.
$ python manage.py startapp api_user
장고에서 app의 개념은 장고 프로젝트 내에서 기능별로 쪼갠 것 이라고 생각하면 된다.
api_user앱은 user에 대한 모든 처리를 담당하는 기능이다.
앱 생성이 정상적으로 완료 되면 다음과 같이 프로젝트 안에 api_user앱이 생성되어 있다.
앱 생성이 완료되면 settings.py에 INSTALLED_APPS에 앱을 추가해 주어야 한다. app 이름 api_user도 추가하면서 앞서 설치한 rest_framework도 함께 추가해준다.
3. REST API 설계
user에 관련된 모든 API는 아래와 같이 제공하기로 한다.
- POST /users 새로운 user정보 생성하기
- GET /users 모든 user에 대한 정보 불러오기
- GET /users/{id} user중 id에 대한 정보 불러오기
- PUT /users/{id} user중 id에 대한 정보 수정하기
- DELETE /users/{id} user중 id에 대한 정보 삭제하기
4. URLConf 설정을 통해 request 라우팅
웹을 통해 장고로 들어온 Reuqest는 아래와 같은 구조로 처리 된다.
- 클라이언트로부터 요청을 받으면 장고는 가장 먼저 최상위 URLConf에서 URL을 분석한다.
이 작업은 프로젝트 django_restapi/urls.py에서 수행한다. (*최상위 URLConf는 settings.py에 ROOT_URLCONF에서 설정한다.) - urls.py에서 api_user앱에 들어온 url이라고 판단하면 요청을 api_user내의 urls.py로 넘긴다.
- api_user/urls.py에서 url을 분석하여 최종적으로 view로 요청을 넘긴다.
- view에서 메소드(CRUD)에 따라 처리를 한다.
django_restapi/urls.py
1
2
3
4
5
6
7
8
9
|
from django.contrib import admin
from django.urls import path
from django.conf.urls import include
urlpatterns = [
path('admin/', admin.site.urls),
path('users/', include('api_user.urls'), name='api_user'), #include 함수를 통해 api_usr의 urls.py로 라우팅 해준다.
]
|
|
|
cs |
api_user/urls.py
1
2
3
4
5
6
7
|
from django.urls import path
from . import views
app_name = 'api_user'
urlpatterns = [
path('', views.UserView.as_view()),#User에 관한 API를 처리하는 view로 Request를 넘김
]
|
cs |
api_user/views.py (APIView에 대해서는 다음 포스트에 다룸)
1
2
3
4
5
6
7
|
from rest_framework.views import APIView
from rest_framework.response import Response
class UserView(APIView):
def get(self, request):
return Response("ok", status=200)#테스트용 Response
|
cs |
runserver를 한 뒤
포스트맨을 통해 아래와 같이 테스트 해보면 정상적으로 응답이 오는걸 확인할 수 있다.
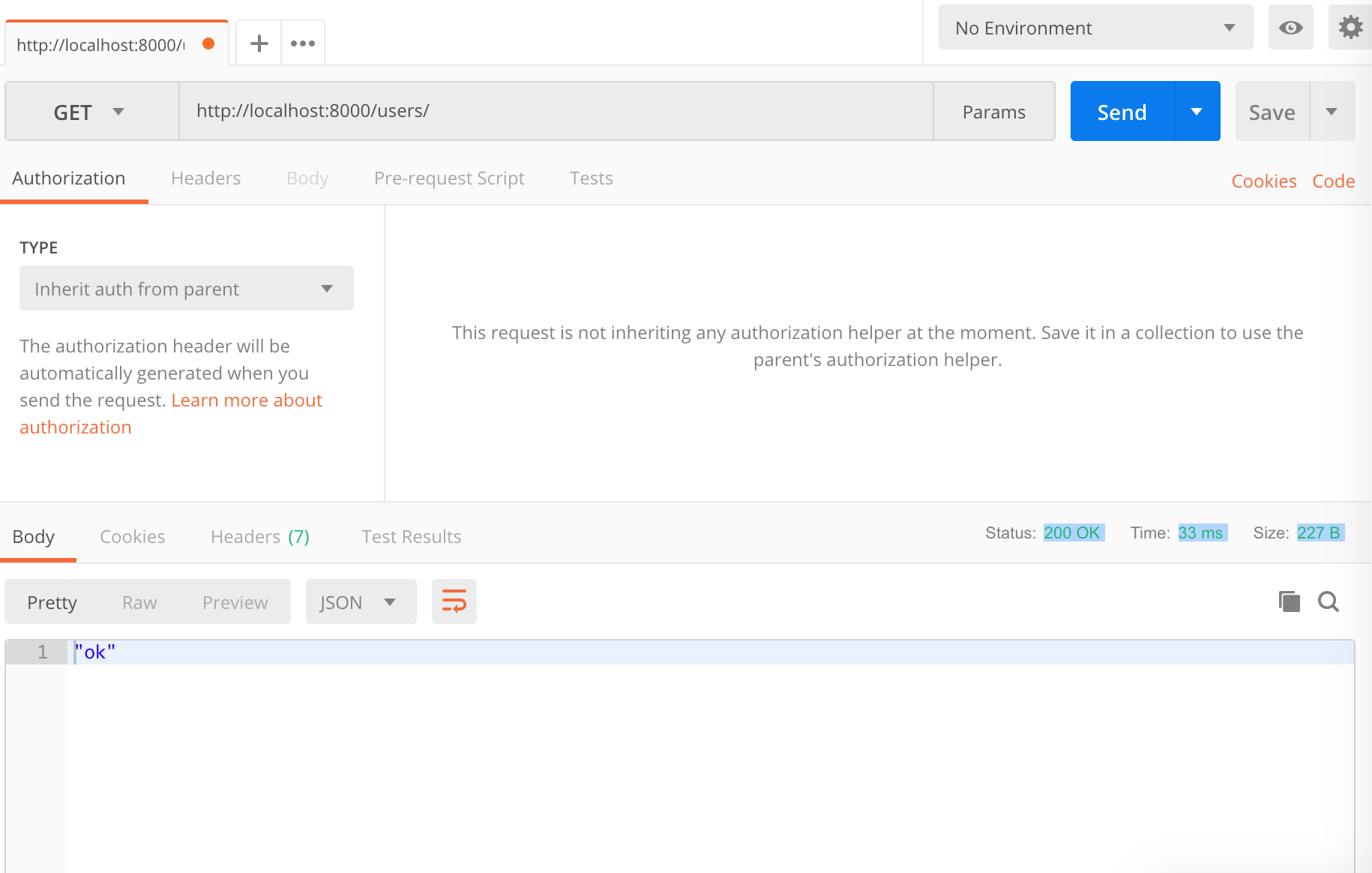
'Python > Django' 카테고리의 다른 글
[Django] [웹 기초] HTTP 기초 (0) | 2020.08.02 |
---|---|
[Django] [웹 기초] 웹 프로그래밍이란 (0) | 2020.07.25 |
[Django] 간단한 REST API 서버 만들기4 - Class Based View에서 POST, GET, PUT, DELETE 구현 (0) | 2020.02.10 |
[Django] 간단한 REST API 서버 만들기3 - model, serializer 구현 (0) | 2020.02.08 |
[Django] 간단한 REST API 서버 만들기1 - 개발 환경설정 (0) | 2020.02.04 |